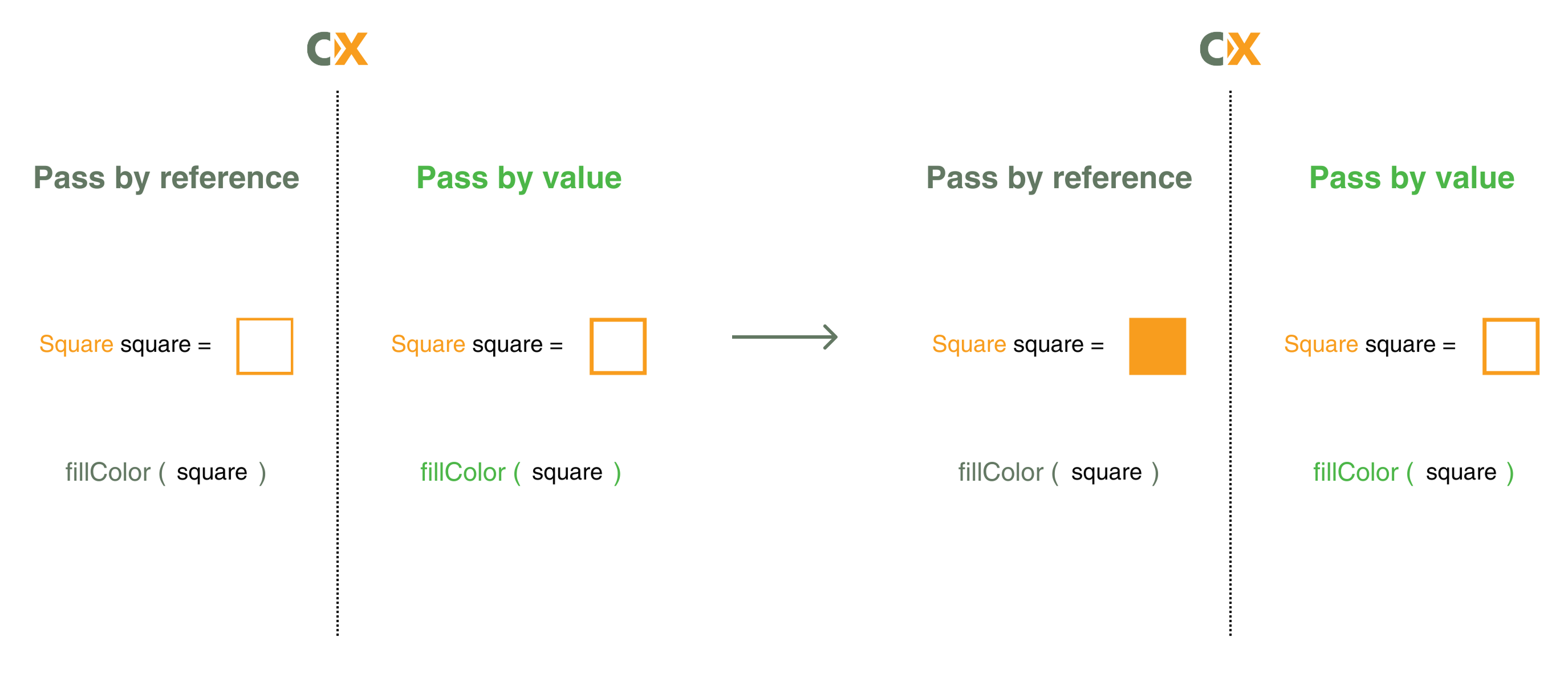
In modern ABAP Object Oriented Programming, we define method parameters using the IMPORTING
, EXPORTING
keywords, each of which can use pass by value or pass by reference, as well as the RETURNING
keyword, which always uses pass by value, and the CHANGING
keyword, which always uses pass by reference.
Let’s understand the difference between them.
1. Pass by Value
When a parameter is passed by value, a copy of the data is made.
This means any changes inside the method do not affect the original variable outside the method. In methods we explicitly define pass by value using the VALUE
keyword.
Example 1) of Pass by Value
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
"Coding CLASS zcl_blog_pass_value_reference DEFINITION PUBLIC FINAL CREATE PUBLIC . PUBLIC SECTION. INTERFACES if_oo_adt_classrun. METHODS change_value IMPORTING i_out TYPE REF TO if_oo_adt_classrun_out VALUE(iv_number) TYPE i. PROTECTED SECTION. PRIVATE SECTION. ENDCLASS. CLASS zcl_blog_pass_value_reference IMPLEMENTATION. METHOD change_value. iv_number = iv_number + 10. "Modifies iv_number, possible because of value( ) despite definition as IMPORTING i_out->write( |Inside Method: { iv_number } | ). "Output: 15 ENDMETHOD. METHOD if_oo_adt_classrun~main. DATA: lo_example TYPE REF TO zcl_blog_pass_value_reference, lv_number TYPE i VALUE 5. lo_example = NEW zcl_blog_pass_value_reference( ). lo_example->change_value( EXPORTING i_out = out iv_number = lv_number ). "Passing the variable by value out->write( |After method: { lv_number }| ). "Output: 5 (Unchanged, because a copy of the value was created and changed) ENDMETHOD. ENDCLASS. |
Explanation:
- The
VALUE
keyword ensuresiv_number
is passed by value, thus a copy of the data is created inside the method. - Any modifications to the copied data within
change_value
do not affectlv_number
outside the method. - Note: The
IMPORTING
parameter was able to be „changed“ due to VALUE( ) without needing to define it as aCHANGING
parameter.
That’s great, but what if instead of:
1 2 |
METHODS change_value IMPORTING VALUE(i_number) TYPE i. |
We have:
1 2 |
METHODS change_value IMPORTING VALUE(i_number) TYPE REF TO i. |
We have IMPORTING VALUE()
, which is implicitly pass-by-value, so it creates a copy of the data, but now we don’t have TYPE i
but TYPE REF TO i
as our type.
Let’s take a look.
Example 2) of Pass by Value
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
"Coding CLASS zcl_blog_pass_value_reference DEFINITION PUBLIC FINAL CREATE PUBLIC . PUBLIC SECTION. INTERFACES if_oo_adt_classrun. METHODS change_value IMPORTING VALUE(iv_number) TYPE REF TO i i_out TYPE REF TO if_oo_adt_classrun_out. PROTECTED SECTION. PRIVATE SECTION. ENDCLASS. CLASS zcl_blog_pass_value_reference IMPLEMENTATION. METHOD change_value. iv_number->* = iv_number->* + 10. "Modifies i_number->*, and is a copy of the reference i_out->write( |Inside Method: { iv_number->* } | ). "Output: 15 ENDMETHOD. METHOD if_oo_adt_classrun~main. DATA: lo_example TYPE REF TO zcl_blog_pass_value_reference, lv_number TYPE i VALUE 5, lo_number TYPE REF TO i. lo_example = NEW zcl_blog_pass_value_reference( ). lo_number = REF #( lv_number ). "Reference to existing variable lo_example->change_value( iv_number = lo_number "Passing the reference, but as pass-by-value i_out = out ). out->write( |After method: { lo_number->* }| ). "Output: 15 (Changed, because variable behind the copy of the same reference (= copy of memory address!) was changed) out->write( |Original lv_number: { lv_number }| ). " Output: 15 (Changed, because variable behind the reference was changed) ENDMETHOD. ENDCLASS. |
Explanation
lv_number
is an integer andlo_number
is a reference that points to its memory location.- The method is pass-by-value and expects a reference, so the reference (memory address) is copied.
- When the dereferenced value behind that address is changed inside the method, the change persists outside the method even on the original
lv_number
since it shares the same address. - Note:
lv_number
->* ue be can be modified, despitelv_number
being desfined as anIMPORTING
parameter
Next, let’s check out pass-by-reference in methods.
2. Pass by Reference
When a parameter is passed by reference, no copy of the data is created.
Instead, the method copies the memory address of the data as a reference and operates directly on the original variable, meaning any changes made inside the method will reflect outside the method, as the original data was changed via this reference.
Example 1) of Pass by Reference
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
"Coding CLASS zcl_blog_pass_value_reference DEFINITION PUBLIC FINAL CREATE PUBLIC . PUBLIC SECTION. INTERFACES if_oo_adt_classrun. METHODS change_reference IMPORTING i_out TYPE REF TO if_oo_adt_classrun_out CHANGING cv_number TYPE i. PROTECTED SECTION. PRIVATE SECTION. ENDCLASS. CLASS zcl_blog_pass_value_reference IMPLEMENTATION. METHOD change_reference. cv_number = cv_number + 10. "Modifies cv_number i_out->write( |Inside Method: { cv_number } | ). "Output: 15 ENDMETHOD. METHOD if_oo_adt_classrun~main. DATA: lo_example TYPE REF TO zcl_blog_pass_value_reference, lv_number TYPE i VALUE 5. lo_example = NEW zcl_blog_pass_value_reference( ). lo_example->change_reference( exporting i_out = out changing cv_number = lv_number ). "Passing the variable by reference out->write( |After method: { lv_number }| ). "Output: 15 (Changed, because the variable behind the reference was changed) ENDMETHOD. ENDCLASS. |
Explanation:
lv_number
is passed by reference to the method, thus the method acts directly on the data behind the reference.- The changes to
cv_number
reflect outside the method onlv_number
. - Note:
cv_number
must be made aCHANGING
parameter, becauseIMPORTING
parameters cannot be changed by reference.
Example 2) of Pass by Reference
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
"Coding CLASS zcl_blog_pass_value_reference DEFINITION PUBLIC FINAL CREATE PUBLIC . PUBLIC SECTION. INTERFACES if_oo_adt_classrun. METHODS change_reference IMPORTING iv_number TYPE REF TO i i_out TYPE REF TO if_oo_adt_classrun_out. PROTECTED SECTION. PRIVATE SECTION. ENDCLASS. CLASS zcl_blog_pass_value_reference IMPLEMENTATION. METHOD change_reference. iv_number->* = iv_number->* + 10. "Modifies i_number->*, and is a refernce to the reference i_out->write( |Inside Method: { iv_number->* } | ). "Output: 15 ENDMETHOD. METHOD if_oo_adt_classrun~main. DATA: lo_example TYPE REF TO zcl_blog_pass_value_reference, lv_number TYPE i VALUE 5, lo_number TYPE REF TO i. lo_example = NEW zcl_blog_pass_value_reference( ). lo_number = REF #( lv_number ). "Reference to existing variable lo_example->change_reference( iv_number = lo_number "Passing the reference to lv_number by reference i_out = out ). out->write( |After method: { lo_number->* }| ). "Output: 15 (Changed, because reference of lo_number (itself a reference) was passed) out->write( |Original lv_number: { lv_number }| ). "Output: 15 (Changed, because lo_number was a reference to lv_number) ENDMETHOD. ENDCLASS. |
Explanation:
lv_number
is an integer andlo_number
is a reference that points to its memory location.- The method is pass-by-reference and expects a reference, so a reference to a reference is acted upon.
- When the dereferenced value behind that reference is changed inside the method, the change persists outside the method even on the original
lv_number
as it was passed by reference
3. Field-Symbols
We’ve pretty much covered pass-by-value and pass-by-reference in the context of methods and their parameters, and we’ve also talked about variables and ref variables.
I know some readers are going to be wondering: “What about Field-Symbols?”
So let’s briefly discuss them as well and you’ll find more details in the performance comparisons below.
What are field-symbols?
Field-symbols are pass-by-reference. Their syntax differs a bit from references, but basically they’re pointers in ABAP, allowing dynamic access to memory locations without copying data or needing to be dereferenced.
While field-symbols offer a slight performance advantage due to not needing to be dereferenced, which is a negligible performance cost, they are becoming obsolete and are not recommended by SAP.
Hence, why I only want to mention them briefly.
4. Compare & Contrast
Pass-by-value vs. Pass-by-reference:
Aspect | Pass-by-value | Pass-by-reference |
Concept | The method receivs a copy of the variables’s value | The method receives a pointer (reference) to the original variable |
Memory Usage | Higher (creates a copy) | Lower (uses original memory) |
Performance | Slower for large data | Faster, especially for tables |
Scope | Changes only affect the copy within the method | Changes affect the original variable directly |
Usage | Common for small data types or when memory is no concern | Common for large structures, itabs and objects |
Data Integrity | Safer (no unintended modifications) | Risk of unintended modifications |
Modifications | No impact on the original data | Modifies the original data directl.y |
Flexibility | Safer, as the original data remains unchanged | More flexible, but requires careful handling |
Syntax | IMPORTING VALUE( ) TYPE | IMPORTING TYPE REF TO |
Field-Symbols vs. References:
Aspect | Field-Symbols (< >) | References (REF #( )) |
Concept | Acts like a pointer, referencing existing memory directly | Creates a reference object pointing to data |
Memory Usage | No new memory allocation | Requires memory for the reference itself |
Performance | Very fast (direct access) | Slightly slower due to dereferencing |
Scope | Only valid within the block where assigned | Persistent across method calls |
Usage | Good for internal table processing, loops, dynamic access | Useful for object-oriented design, method parameters |
Modifications | Changes the original data directly | Changes data via the reference |
Flexibility | More flexible for direct memory operations | Better for passing objects and structured data |
Dereferencing | Implicit (no extra step needed) | Explicit (lo_ref->*) |
*Dereferencing is very fast, operating at memory access speed. The performance cost is negligible compared to large data copies or database accesses.
5. Overview of Behaviour
- In ABAP OOP, using
VALUE()
or not in yourIMPORTING
andEXPORTING
parameters determines whether the method implicitly behaves as pass-by-value or pass-by-reference. - A reference to an object is the memory address of an object via which the object can be accessed (dereferenced, ->*) and acted upon. Passing only memory addresses requires less computation than copying the entire datasets around.
- Pass-by-value methods create a copy of the object, even when the object itself is a reference. Thus the original object stays unchanged outside the method.
- Pass-by-reference methods access the memory address of the original object and may act upon its data by dereferencing. The original object doesn’t stay unchanged outside the method. This can sometimes lead to unexpected behavior, when appending to
EXPORTING
tables in separate method calls throughout a program, where one would expect the parameter to be cleared automatically with each call, but isn’t. Manual clearing ofEXPORTING
parameters for tables, is often wanted, when appending to pass-by-reference parameters. CHANGING
is always pass-by-reference andRETURNING
is always pass-by-value.IMPORTING
andEXPORTING
can be either.
Conclusion
Modern ABAP OOP syntax allows clear control over pass by reference vs. pass by value, influencing performance and data integrity. By understanding these principles, developers can write more efficient and maintainable code in ABAP.
Let’s Keep the Conversation Going!
Do you have questions or your own experiences on this topic? Feel free to reach out to Jonathan Rumpl on LinkedIn!
Stay up to date! Sign up for our Cadaxo newsletter and receive regular insights, best practices, and news from the SAP world.